how to draw a 3d hammer
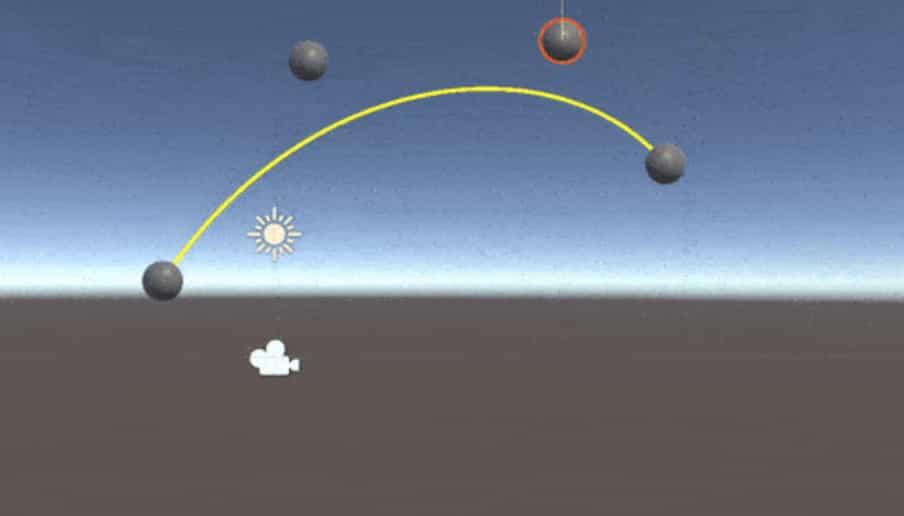
Sometimes, you need to draw lines, circles or curves in your Unity games. In these cases, you can use Unity's LineRenderer class. In this tutorial, we will come across how nosotros can draw lines, polygons, circles, wave functions, Bézier Curves. And also nosotros will see how we can do a free drawing using Line Renderer in Unity3D. In order to come across our other Unity tutorials, click hither.
Line Renderer Component
To draw a line we have to add a LineRenderer component to a game object. Even if this component can be attached to whatever game object, I suggest you lot create an empty game object and add the LineRenderer to this object.
We demand a material which volition be assigned to LineRenderer. To practise this create a material for the line in Projection Tab. Unlit/Color shader is suitable for this material.
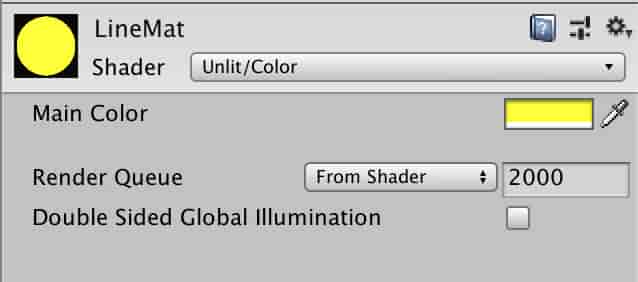
Assign LineMat fabric to the Line Renderer component.
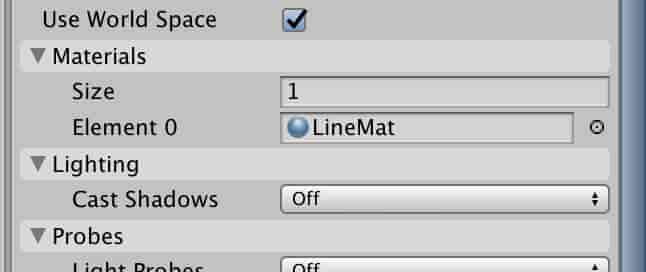
Line Renderer draws lines between determined positions. In other words, nosotros tell the Line Renderer the points which will exist connected and Line Renderer connects these points.
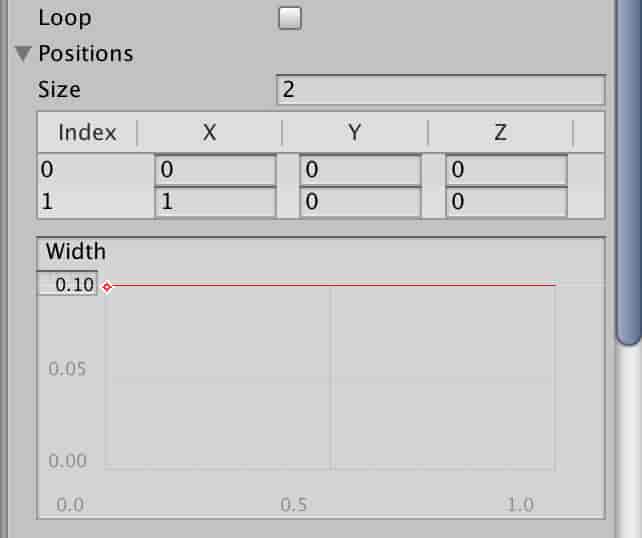
In the Positions section, y'all tin can change the number of points and positions of points. If you lot enter two different points, y'all will get a straight line. You can also change the width of the line in the department below.
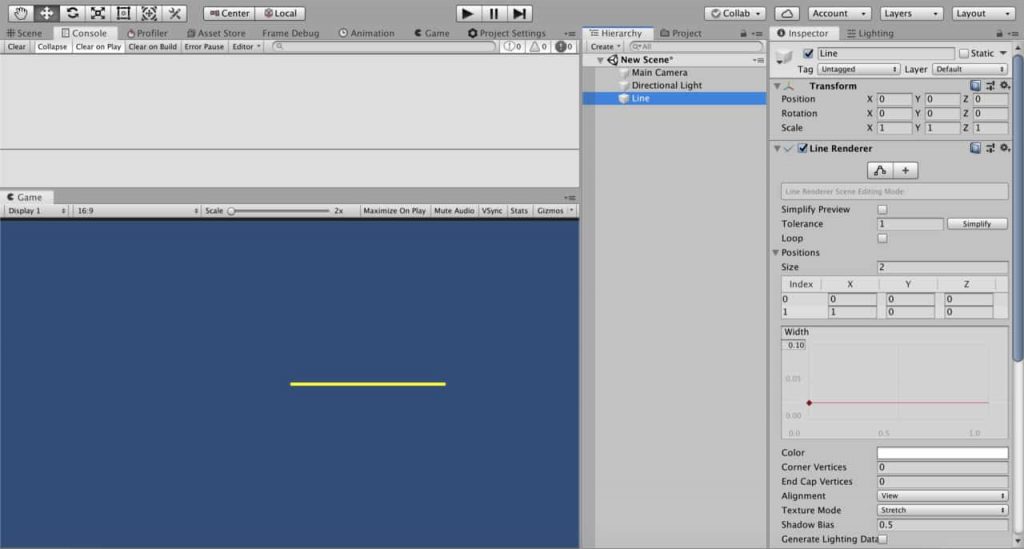
Likewise, 2 depict a triangle, you lot demand 3 points and to draw a rectangle you need 4 points. Allow'due south draw a rectangle equally an instance.
To describe a rectangle, nosotros need to set positions of 4 points. We too have to check the Loop toggle to obtain a closed shape.
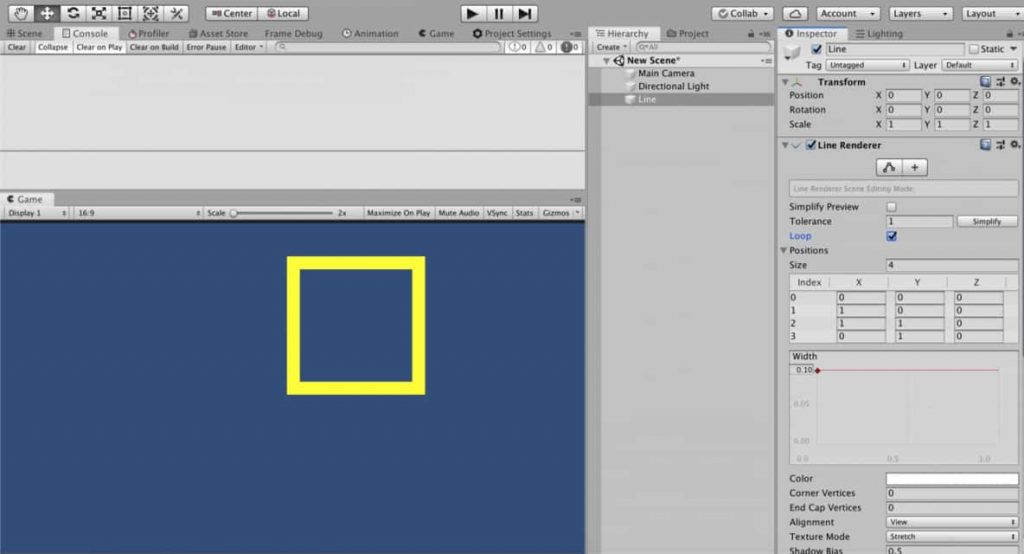
Drawing Lines From C# Script
If we want to draw or control lines in existent-fourth dimension, nosotros demand to create a C# script. To describe lines from a script, we determine the size of position array and coordinates of positions in C# script. Therefore, LineRenderer tin can connect the points.
Permit's describe a triangle using a script as an case. Showtime, create a script with the name "DrawScript". And attach this script to a game object which already has a LineRenderer component.
public grade DrawScript : MonoBehaviour { private LineRenderer lineRenderer; void Start( ) { lineRenderer = GetComponent<LineRenderer> ( ) ; Vector3[ ] positions = new Vector3[ 3 ] { new Vector3( 0 , 0 , 0 ) , new Vector3( - 1 , 1 , 0 ) , new Vector3( one , 1 , 0 ) } ; DrawTriangle(positions) ; } void DrawTriangle(Vector3[ ] vertexPositions) { lineRenderer.positionCount = three ; lineRenderer.SetPositions(vertexPositions) ; } }
This script volition draw a triangle. Note that we already ready the line width to 0.ane and checked the loop toggle, before. Therefore the same setting is also valid here.
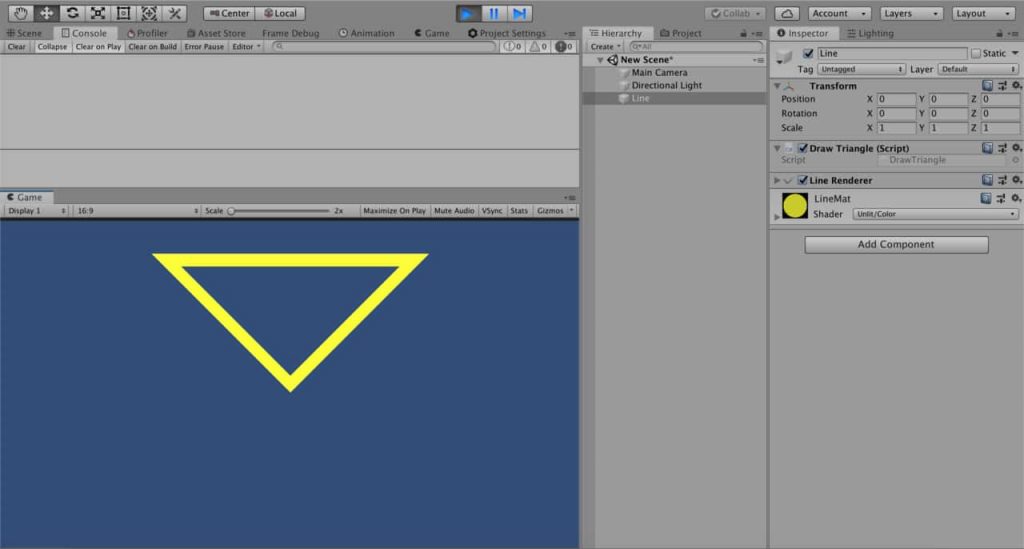
We can also change the line width from the script using startWidth and endWidth. In addition to this, if you would like to change line width by position, yous tin can prepare different values to them. In this case, Line Renderer will interpolate the line width according to position.
public class DrawScript : MonoBehaviour { private LineRenderer lineRenderer; void Start( ) { lineRenderer = GetComponent<LineRenderer> ( ) ; Vector3[ ] positions = new Vector3[ iii ] { new Vector3( 0 , 0 , 0 ) , new Vector3( - 1 , 1 , 0 ) , new Vector3( 1 , 1 , 0 ) } ; DrawTriangle(positions, 0.02 f , 0.02 f ) ; } void DrawTriangle(Vector3[ ] vertexPositions, float startWidth, float endWidth) { lineRenderer.startWidth = startWidth; lineRenderer.endWidth = endWidth; lineRenderer.loop = truthful ; lineRenderer.positionCount = 3 ; lineRenderer.SetPositions(vertexPositions) ; } }
Drawing Regular Polygons and Circles
In this section, nosotros are going to see how we tin can write a method that draws regular polygons. Since circles are n-gons which has big northward, our function will be useful for circles as well. But start, let me explicate the mathematics behind it.
Vertices of regular polygons are on a circle. Also, the centre of the circumvolve and the heart of the polygon are pinnacle of each other. The most reliable method to draw a polygon is to find the bending between successive vertices and locate the vertices on the circle. For example, angle of the arc between successive vertices of a pentagon is 72 degrees or for an octagon, information technology is 45 degrees. To find this angle, we can divide 360 degrees(or 2xPI radians) with the number of vertices.
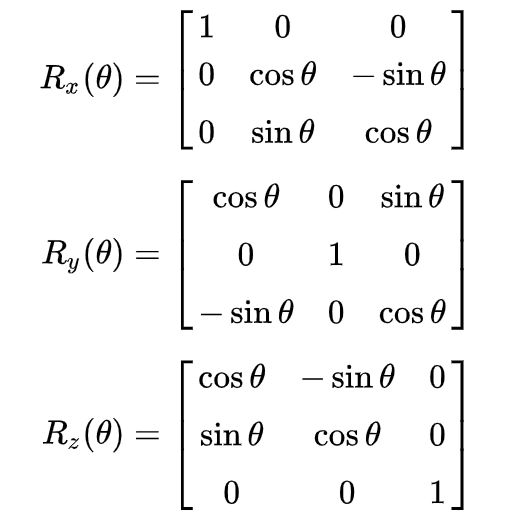
Then we need to notice the positions of the vertices. To do this we assign an initial point for the start vertex and rotate this vertex for each vertex using a rotation matrix.
Every bit y'all probably know, in order to rotate a indicate around an axis, we multiply the position vector of the bespeak with the rotation matrix. Rotation matrices for rotations around 10, y and z axes are given on the correct.
For example, when we want to rotate a point by 90 degrees around the z-centrality, which has a coordinate (1,0,0), we multiply the position vector past a rotation matrix.

Nosotros need to construct a rotation matrix to rotate each vertex around the z-centrality. Permit's me write our DrawPolygon method first and explain it.
void DrawPolygon( int vertexNumber, bladder radius, Vector3 centerPos, float startWidth, float endWidth) { lineRenderer.startWidth = startWidth; lineRenderer.endWidth = endWidth; lineRenderer.loop = truthful ; float angle = 2 * Mathf.PI / vertexNumber; lineRenderer.positionCount = vertexNumber; for ( int i = 0 ; i < vertexNumber; i+ + ) { Matrix4x4 rotationMatrix = new Matrix4x4( new Vector4(Mathf.Cos(bending * i) , Mathf.Sin(angle * i) , 0 , 0 ) , new Vector4( - 1 * Mathf.Sin(bending * i) , Mathf.Cos(angle * i) , 0 , 0 ) , new Vector4( 0 , 0 , i , 0 ) , new Vector4( 0 , 0 , 0 , 1 ) ) ; Vector3 initialRelativePosition = new Vector3( 0 , radius, 0 ) ; lineRenderer.SetPosition(i, centerPos + rotationMatrix.MultiplyPoint(initialRelativePosition) ) ; } }
Y'all may wonder why the constructed rotation matrix is 4×four. In computer graphics, the 3-dimensional world is represented every bit 4-dimensional simply this topic is non related to our business hither. We simply utilise information technology every bit if it is three-dimensional.
We set the position of initial vertex and rotate it using rotationMatrix each time and add the center position to it.
The post-obit image is an case of a hexagon which is drawn by this method.
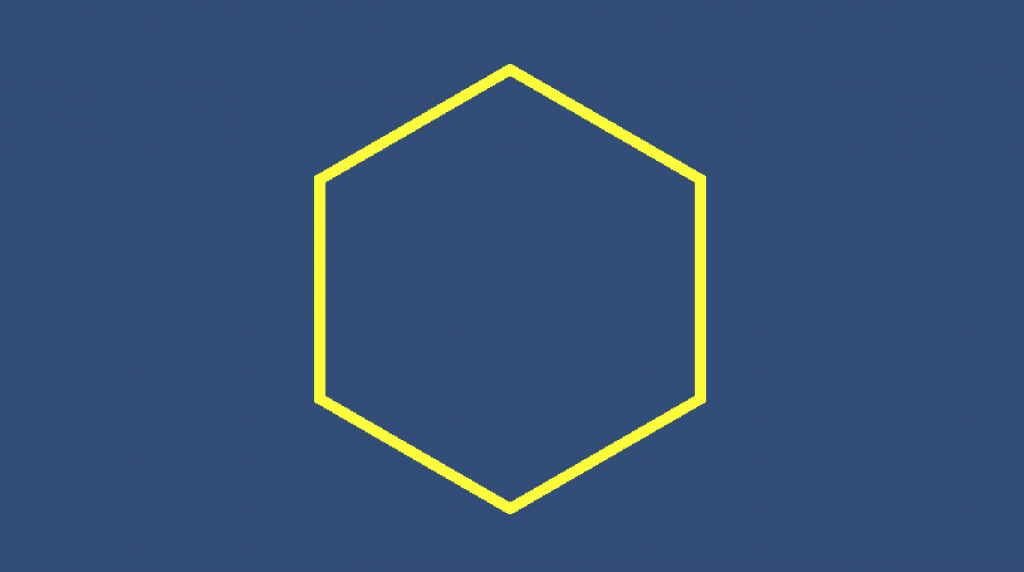
If you increase the number of vertex points, this polygon turns to a circle.
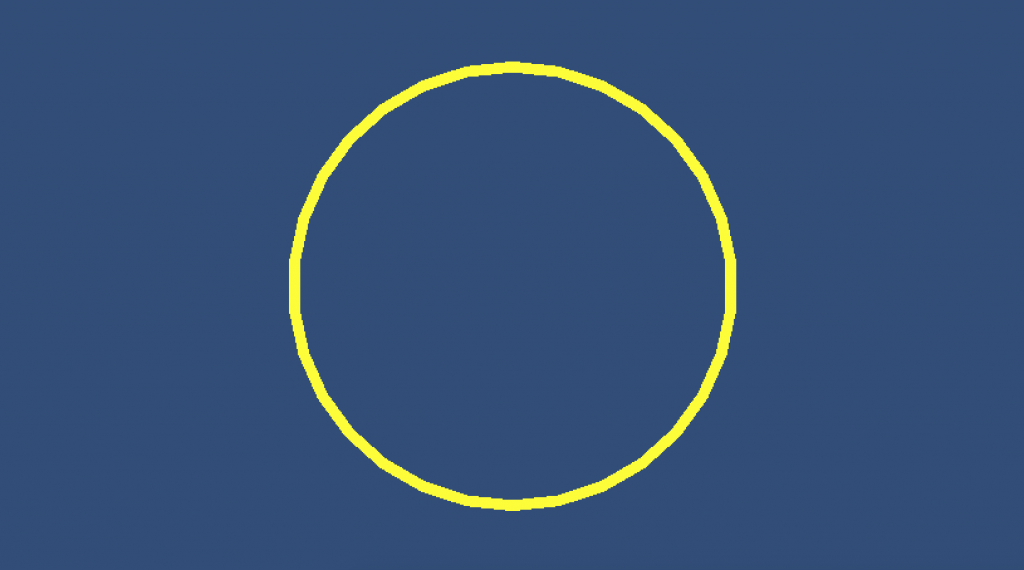
Drawing Waves
In this section, nosotros are going to draw a sinusoidal wave, a traveling wave and a continuing moving ridge using sine function.
The mathematical role of the sine wave is given by the following:
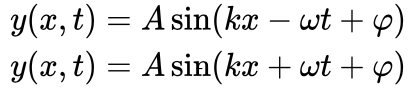
where
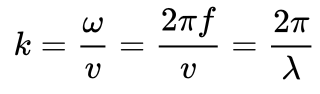
Here, k is wave number, f is frequency, ω is the angular frequency, λ is wavelength, 5 is the linear speed, t is the time and φ is the stage angle. We will not worry about the phase angle in our discussions.
Sine moving ridge equation with a minus sign represents traveling moving ridge from left to right and the equation with plus sign represents a traveling line wave correct to left.
In society to draw a stable sinusoidal moving ridge, we can drop the time part. The following method will describe a sine wave.
void DrawSineWave(Vector3 startPoint, bladder amplitude, float wavelength) { float x = 0f; float y; float yard = 2 * Mathf.PI / wavelength; lineRenderer.positionCount = 200 ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { x + = i * 0.001 f ; y = amplitude * Mathf.Sin(one thousand * x) ; lineRenderer.SetPosition(i, new Vector3(x, y, 0 ) + startPoint) ; } }
Our DrawSineWave method takes three parameters. They are startPoint which is for setting the start position in world space, amplitude which is for setting the amplitude of the wave and wavelength which is for setting the wavelength of the sine wave.
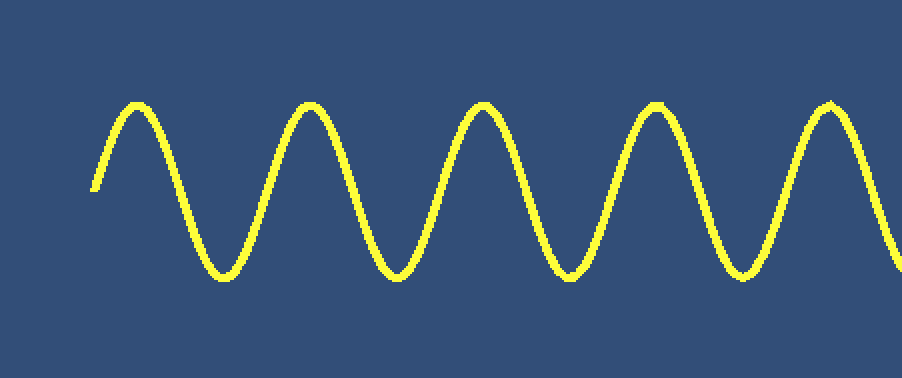
To obtain the positions of the respective mathematical function, first, we make up one's mind the positions on the 10-centrality. For each ten, we accept to calculate the y-position.
To animate this wave, nosotros have to implement time to our function as follows:
void DrawTravellingSineWave(Vector3 startPoint, bladder amplitude, bladder wavelength, float waveSpeed) { float 10 = 0f; float y; float thou = 2 * Mathf.PI / wavelength; float w = m * waveSpeed; lineRenderer.positionCount = 200 ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { x + = i * 0.001 f ; y = amplitude * Mathf.Sin(k * x + due west * Time.time) ; lineRenderer.SetPosition(i, new Vector3(x, y, 0 ) + startPoint) ; } }
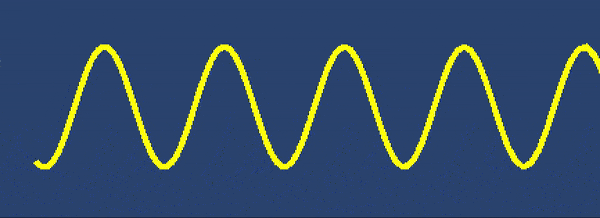
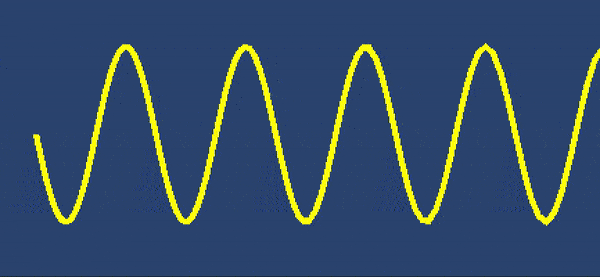
This fourth dimension we have four parameters. The fourth parameter is to gear up the moving ridge speed. This moving ridge travels to the left since we used plus sign in the function. If we would similar to create a moving ridge that travels to the right, we have to utilise the minus sign. Yous should keep in heed that nosotros take to write this method in Update().
To create a continuing wave, we take to add two waves which travel to the right and which travel to left.
void DrawStandingSineWave(Vector3 startPoint, float amplitude, float wavelength, bladder waveSpeed) { bladder 10 = 0f; float y; float k = 2 * Mathf.PI / wavelength; float w = k * waveSpeed; lineRenderer.positionCount = 200 ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { x + = i * 0.001 f ; y = amplitude * (Mathf.Sin(grand * x + w * Time.fourth dimension) + Mathf.Sin(m * 10 - w * Time.time) ) ; lineRenderer.SetPosition(i, new Vector3(10, y, 0 ) + startPoint) ; } }
Cartoon Bézier Curves
Bézier curves are parametric curves that are used to create smooth curved shapes. They are widely used in computer graphics. In this section, we are going to see how nosotros can draw Bézier curves.
When a Bézier bend is controlled by 3 points, and then information technology is called Quadratic Bézier Curve(the showtime equation below) and when information technology is controlled by 4 points, it is chosen Cubic Bézier Bend.


The post-obit script will draw a quadratic Bézier curve using positions p0, p1, and p2. Yous should create three game objects and assign these objects to corresponding variables in the script to change the shape of the bend in real-fourth dimension.
using Organization.Collections; using System.Collections.Generic; using UnityEngine; public class BezierScript : MonoBehaviour { private LineRenderer lineRenderer; public Transform p0; public Transform p1; public Transform p2; void Get-go( ) { lineRenderer = GetComponent<LineRenderer> ( ) ; } void Update( ) { DrawQuadraticBezierCurve(p0.position, p1.position, p2.position) ; } void DrawQuadraticBezierCurve(Vector3 point0, Vector3 point1, Vector3 point2) { lineRenderer.positionCount = 200 ; float t = 0f; Vector3 B = new Vector3( 0 , 0 , 0 ) ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { B = ( i - t) * ( 1 - t) * point0 + 2 * ( 1 - t) * t * point1 + t * t * point2; lineRenderer.SetPosition(i, B) ; t + = ( 1 / ( float )lineRenderer.positionCount) ; } } }
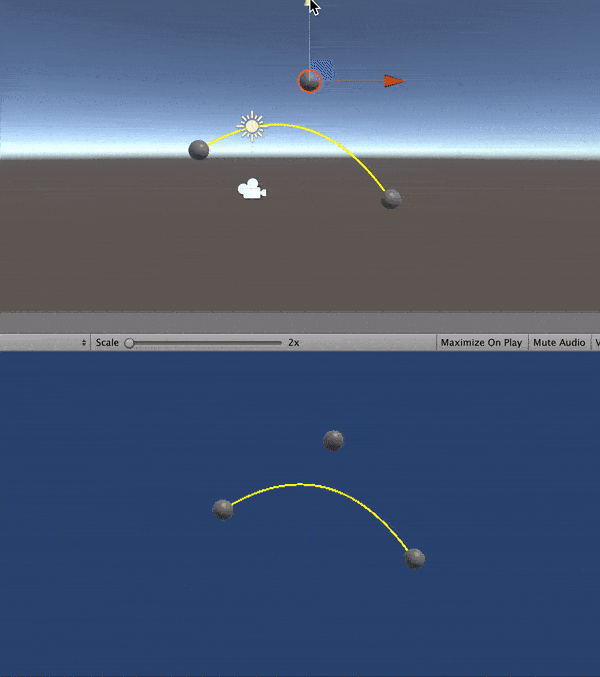
Too, the post-obit method draws a cubic Bézier curve. This fourth dimension we need 4 points.
void DrawCubicBezierCurve(Vector3 point0, Vector3 point1, Vector3 point2, Vector3 point3) { lineRenderer.positionCount = 200 ; bladder t = 0f; Vector3 B = new Vector3( 0 , 0 , 0 ) ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { B = ( 1 - t) * ( 1 - t) * ( 1 - t) * point0 + 3 * ( ane - t) * ( ane - t) * t * point1 + 3 * ( i - t) * t * t * point2 + t * t * t * point3; lineRenderer.SetPosition(i, B) ; t + = ( 1 / ( float )lineRenderer.positionCount) ; } }
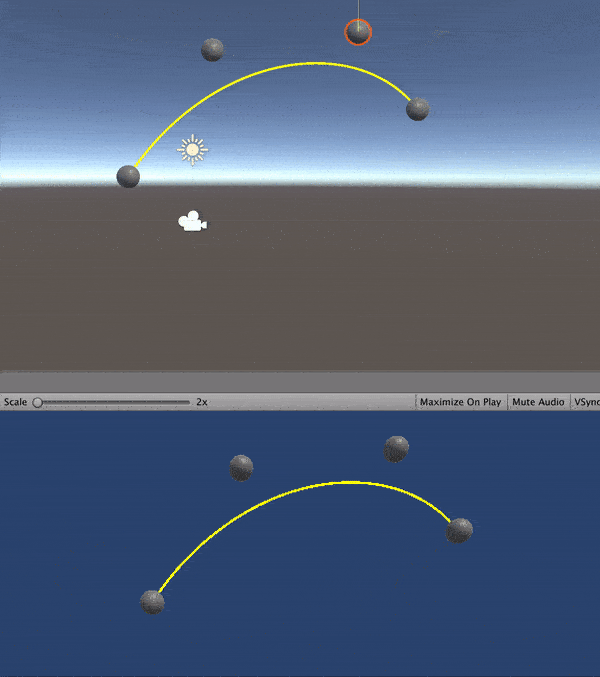
Free Drawing using Line Renderer
In this section, we are going to see how we can draw freely using the mouse position. We can practice this past creating a new game object with a line renderer attached. When we press the left mouse button, a new game object is created and each frame the position of the mouse added to the line renderer.

Beginning of all, we need a prefab to create a new game object when nosotros printing the left mouse button. This is an empty game object with a line renderer component attached. In addition to this, do not forget to assign a fabric to the line renderer component. Create a prefab from this game object.
Second, create an empty game object and adhere the following script DrawManager.
using Arrangement.Collections; using System.Collections.Generic; using UnityEngine; public class DrawManager: MonoBehaviour { private LineRenderer lineRenderer; public GameObject drawingPrefab; void Update( ) { if (Input.GetMouseButtonDown( 0 ) ) { GameObject drawing = Instantiate(drawingPrefab) ; lineRenderer = drawing.GetComponent<LineRenderer> ( ) ; } if (Input.GetMouseButton( 0 ) ) { FreeDraw( ) ; } } void FreeDraw( ) { lineRenderer.startWidth = 0.1 f ; lineRenderer.endWidth = 0.1 f ; Vector3 mousePos = new Vector3(Input.mousePosition.x, Input.mousePosition.y, 10f) ; lineRenderer.positionCount+ + ; lineRenderer.SetPosition(lineRenderer.positionCount - 1 , Photographic camera.main.ScreenToWorldPoint(mousePos) ) ; } }
When you press the left mouse button, a new game object is instantiated from the prefab which we created before. We get the line renderer component from this game object. And so while we are pressing the left mouse button, we call FreeDraw() method.
In the FreeDraw method, we take x and y components of the mouse position and set the z-position equally 10. Here, the mouse position is in the screen space coordinates. But we utilise world space coordinates in line renderer. Therefore we need to convert mouse position to earth space coordinates. In each frame, nosotros too need to increase the number of points. Since nosotros do non know how many points we demand, we cannot fix position count before.
References
1-https://docs.unity3d.com/ScriptReference/LineRenderer.html
two-http://www.theappguruz.com/blog/bezier-bend-in-games
3-https://en.wikipedia.org/wiki/Bézier_curve
iv-https://en.wikipedia.org/wiki/Sine_wave
Source: https://www.codinblack.com/how-to-draw-lines-circles-or-anything-else-using-linerenderer/
0 Response to "how to draw a 3d hammer"
Post a Comment